Mastering Async JavaScript: A Senior-Level Interview Challenge 🚀
JavaScript enthusiasts đź‘‹! Have you ever encountered JavaScript interview questions that test not just your coding skills but also your understanding of how JavaScript really works under the hood?
Today, I’ll walk you through a fascinating code snippet often posed in senior developer interviews. By the end of this post, you'll not only understand the execution flow but also gain practical insights to explain it confidently.
Ready? Let’s dive in! ⚡
The Interview Challenge: The Code
Consider the following JavaScript code snippet. Can you predict the output?
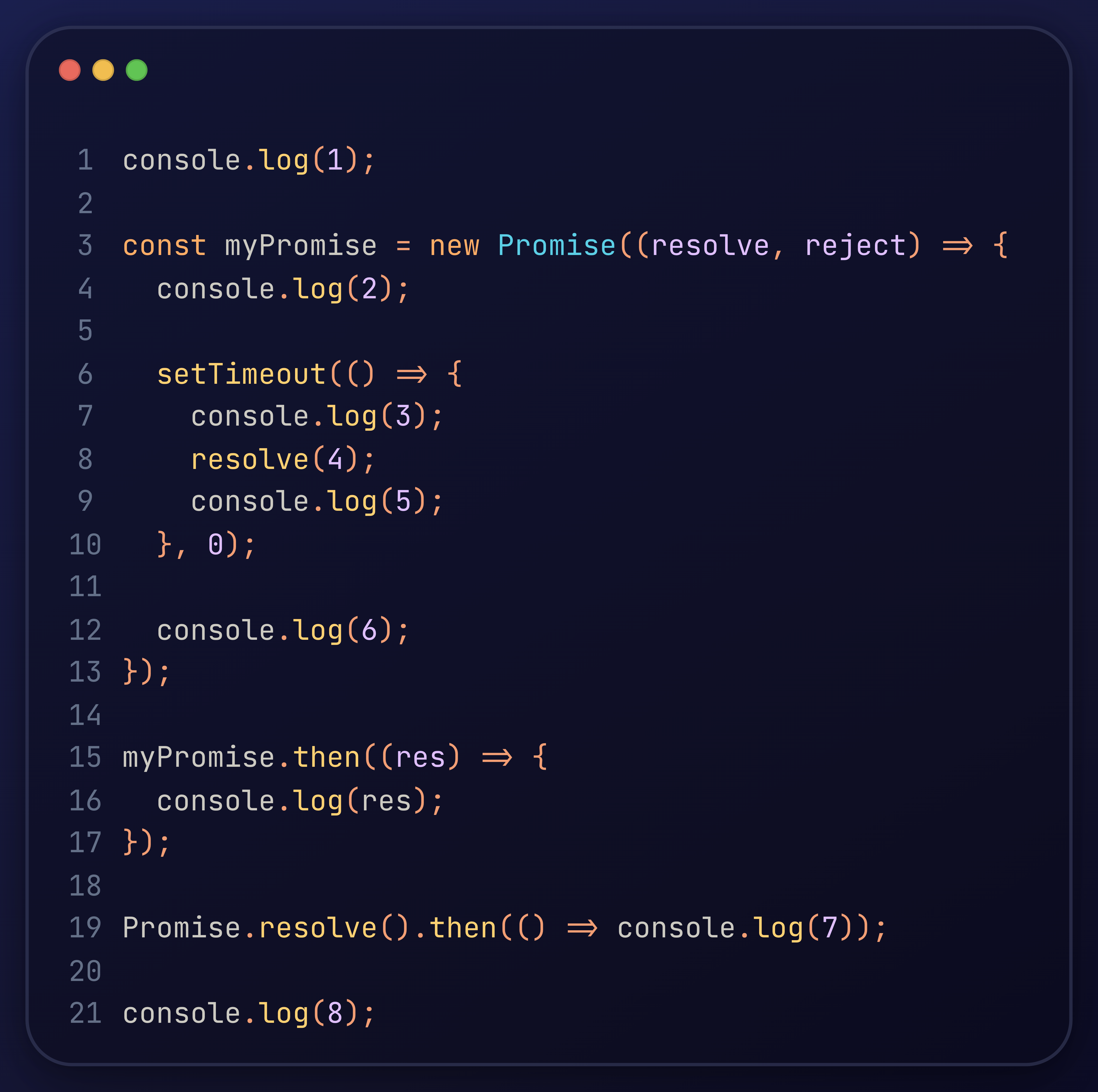
Predicting the Output
At first glance, this code might seem confusing. But take a deep breath—this is just a great opportunity to flex your knowledge of JavaScript’s event loop, microtasks, and macrotasks.
Here’s the actual output:
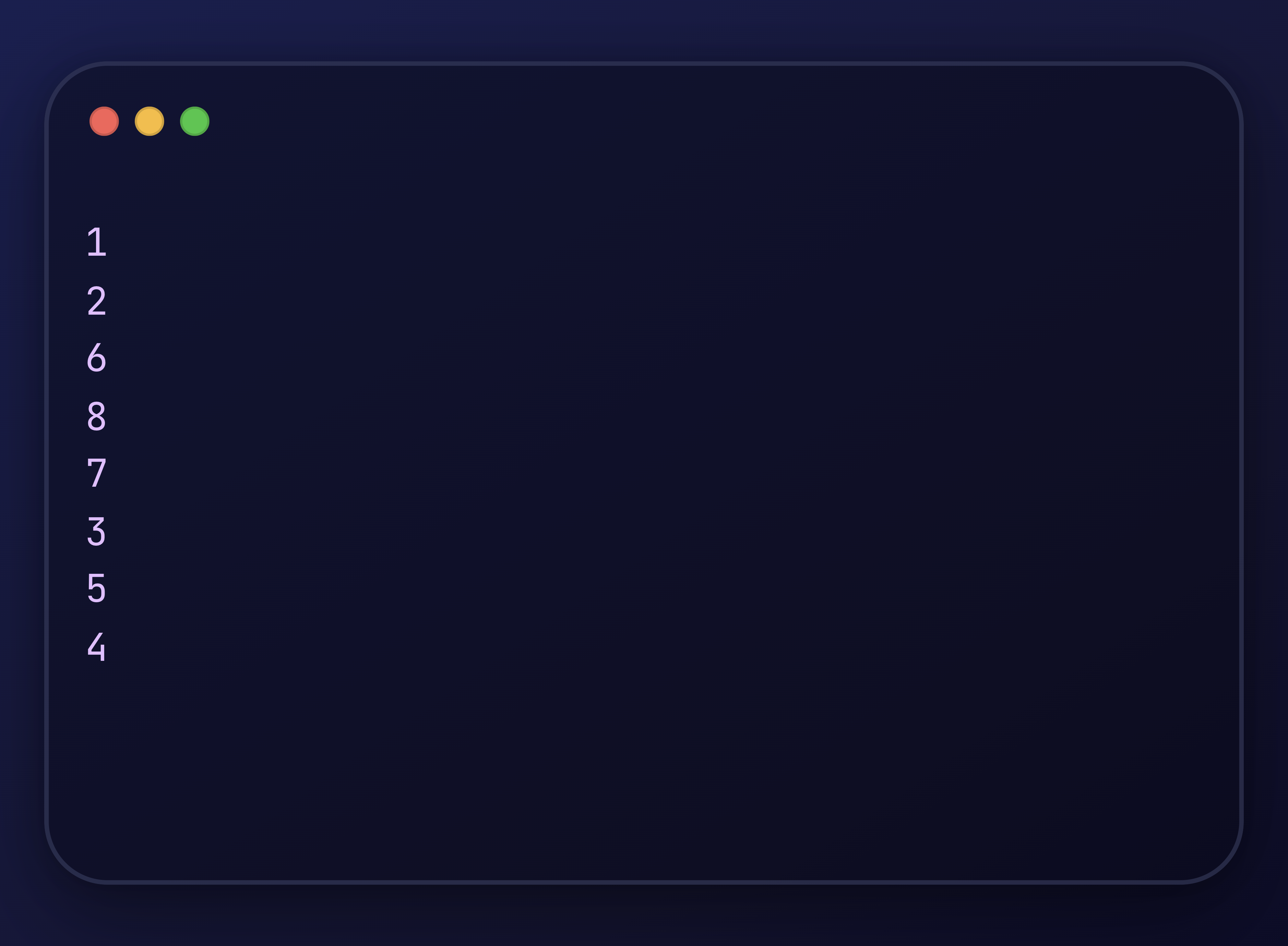
🤔 Did you guess it correctly? Don’t worry if you didn’t; let's break it all down, step by step.
Breaking It Down: Understanding the Execution Order 🚀
To solve this challenge, you need to understand the synchronous execution, the event loop, and the role of microtasks (like Promise.then()
) versus macrotasks (such as setTimeout
).
1. Synchronous Execution First
The javascript call stack always runs synchronous code first. Let’s follow the sync operations:
console.log(1)
executes immediately → Output: 1- The
Promise
executor runs synchronously upon creation:console.log(2)
executes → Output: 2setTimeout()
schedules a macrotask (deferred for later).console.log(6)
executes → Output: 6
- Finally,
console.log(8)
executes after the promise is created → Output: 8
At this point, the synchronous code has completed execution.
2. Microtasks Queue (e.g. Promises) Come Next
Now, the event loop moves to the microtasks queue. Promises and their .then()
handlers are always prioritized over macrotasks.
Two microtasks are queued:
myPromise.then()
Promise.resolve().then()
The second microtask executes first because myPromise
isn't resolved yet
Promise.resolve().then(() => console.log(7))
resolves immediately and logs → Output: 7
3. Macrotasks (e.g. Timers) Are Last
After completing microtasks, the event loop moves to the macrotasks queue. The setTimeout()
callback runs:
console.log(3)
executes → Output: 3- Inside the timer callback:
resolve(4)
triggers the promise. Although the promise resolves, its.then()
handler is queued as a microtask and won’t execute immediately.console.log(5)
executes → Output: 5
Finally, the .then()
handler for the resolved promise (myPromise) runs its microtask:
console.log(res)
executes withres = 4
→ Output: 4
The Event Loop Explained 🔄
To recap, here’s the order of operation considering the call stack, microtask queue, and macrotask queue:
- Call Stack executes synchronous code first
- Regular console.logs →
1, 2, 6, 8
- Regular console.logs →
- Microtasks Queue (Promise callbacks) executes next
- Promise.resolve().then() →
7
- Promise.resolve().then() →
- Macrotasks Queue (setTimeout, setInterval) executes last
- setTimeout callback →
3, 5
- setTimeout callback →
- Resolved Promise microtask →
4
Pro Tips for Understanding Async JavaScript 🎯
If you’d like to feel more confident when handling async interview questions, keep these insights in mind:
Promise Executors Run Synchronously
When you create a new
Promise
, its executor (the function passed in the constructor) is run synchronously. Deferred actions likeresolve()
andreject()
only take effect when queued as microtasks. So, Promise executors run synchronously, but their callbacks are microtasks.- Microtasks vs. Macrotasks
- Microtasks (like
Promise.then()
) always run before macrotasks. - Macrotasks (like
setTimeout
) only run after the microtasks queue is empty.
- Microtasks (like
Code After
resolve()
Executes NormallyAfter calling
resolve()
inside a Promise, the subsequent code within the executor function still runs synchronously before the microtask triggers.
Practical Applications and Key Takeaways 🛠️
Understanding async behavior isn’t just about acing interviews. It's crucial for building performant, responsive web applications 🔥. Here are some practical uses:
- When working with APIs, Promise chaining ensures proper sequencing of operations.
- setTimeout() is frequently used for lightweight task scheduling but must be handled carefully to avoid race conditions.
- Knowing microtask prioritization can prevent unexpected behaviors when mixing Promises and timers.
Conclusion
Understanding JavaScript's event loop and task queuing is crucial for writing efficient, bug-free code. Keep practicing these concepts, and you'll ace those technical interviews!
Happy coding! 🚀